flatMap
flatMap
Stream("abc")
.flatMap { str =>
Stream.emits(str.toList)
}
.compile
.toList

flatMap (with chunks)
Stream("abc")
.flatMap { str =>
Stream.emits(str.toList)
}
.compile
.toList

error propagation
Stream("abc")
.flatMap { _ =>
Stream.raiseError[IO](Err)
}
.compile
.toList
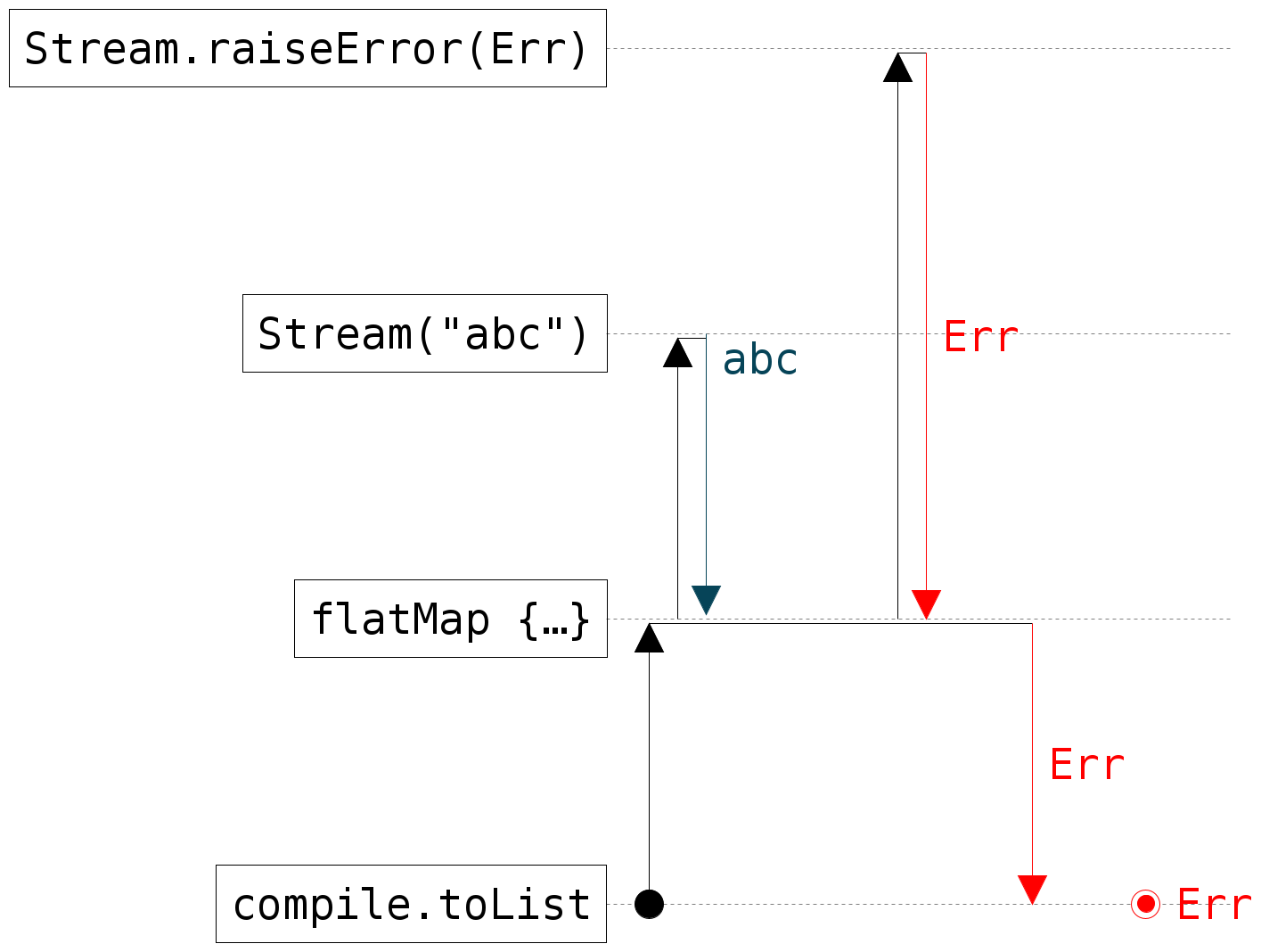
error propagation (with chunks)
Stream("abc")
.flatMap { _ =>
Stream.raiseError[IO](Err)
}
.compile
.toList
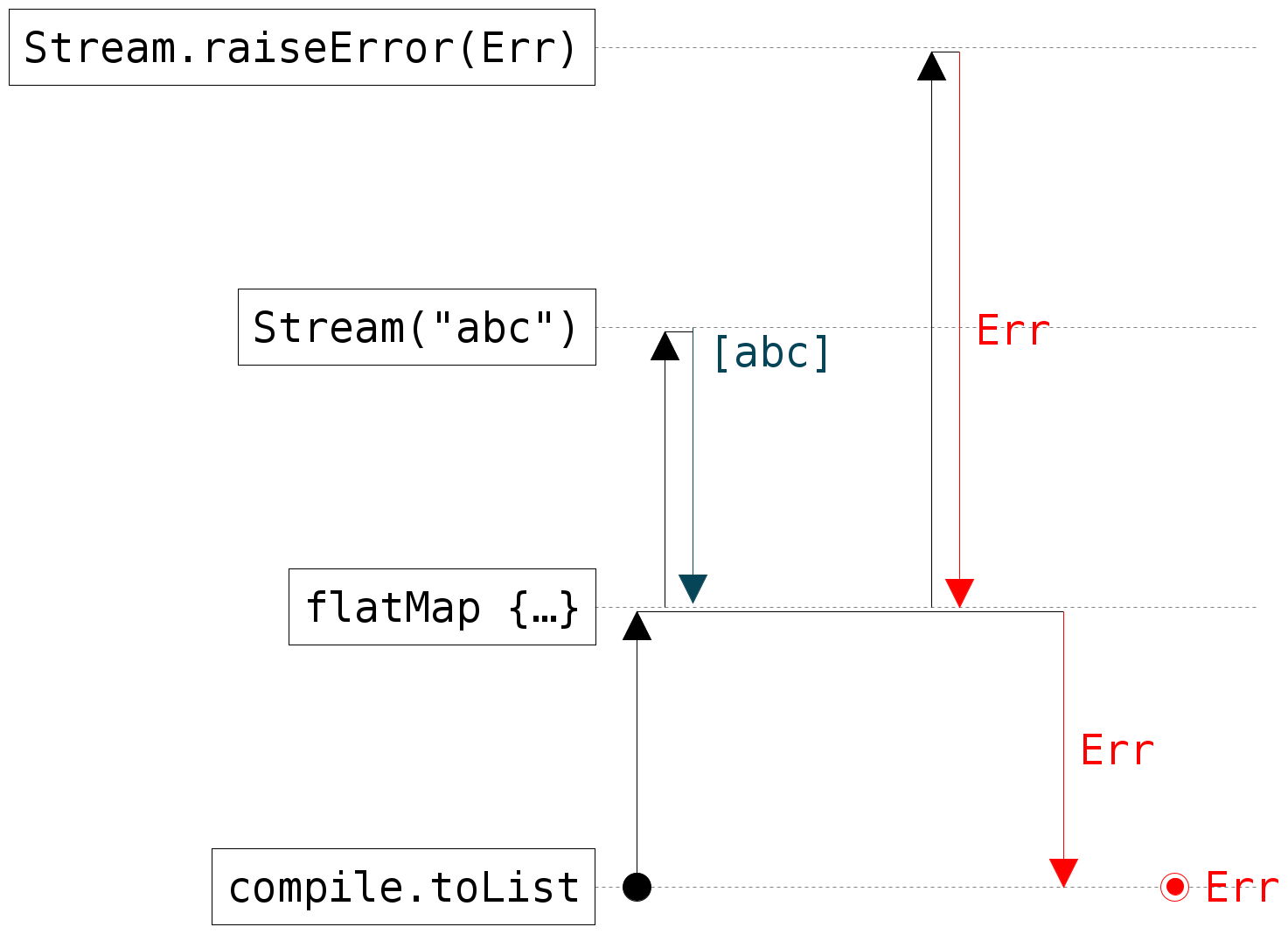
error handling
Stream("abc")
.flatMap { _ =>
Stream.raiseError[IO](Err)
}
.handleError(_ => 'a')
.compile
.toList
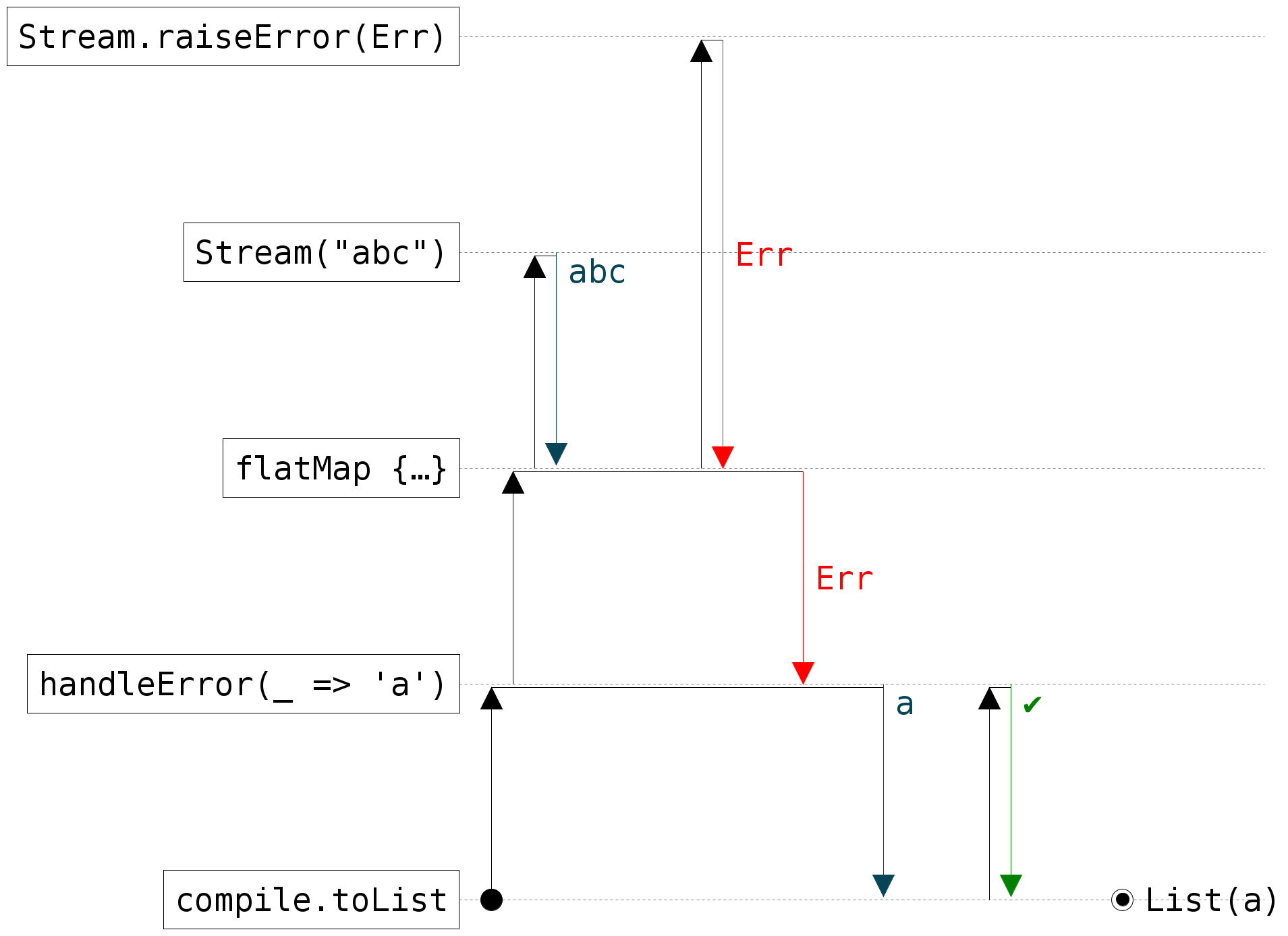
error handling (with chunks)
Stream("abc")
.flatMap { _ =>
Stream.raiseError[IO](Err)
}
.handleError(_ => 'a')
.compile
.toList
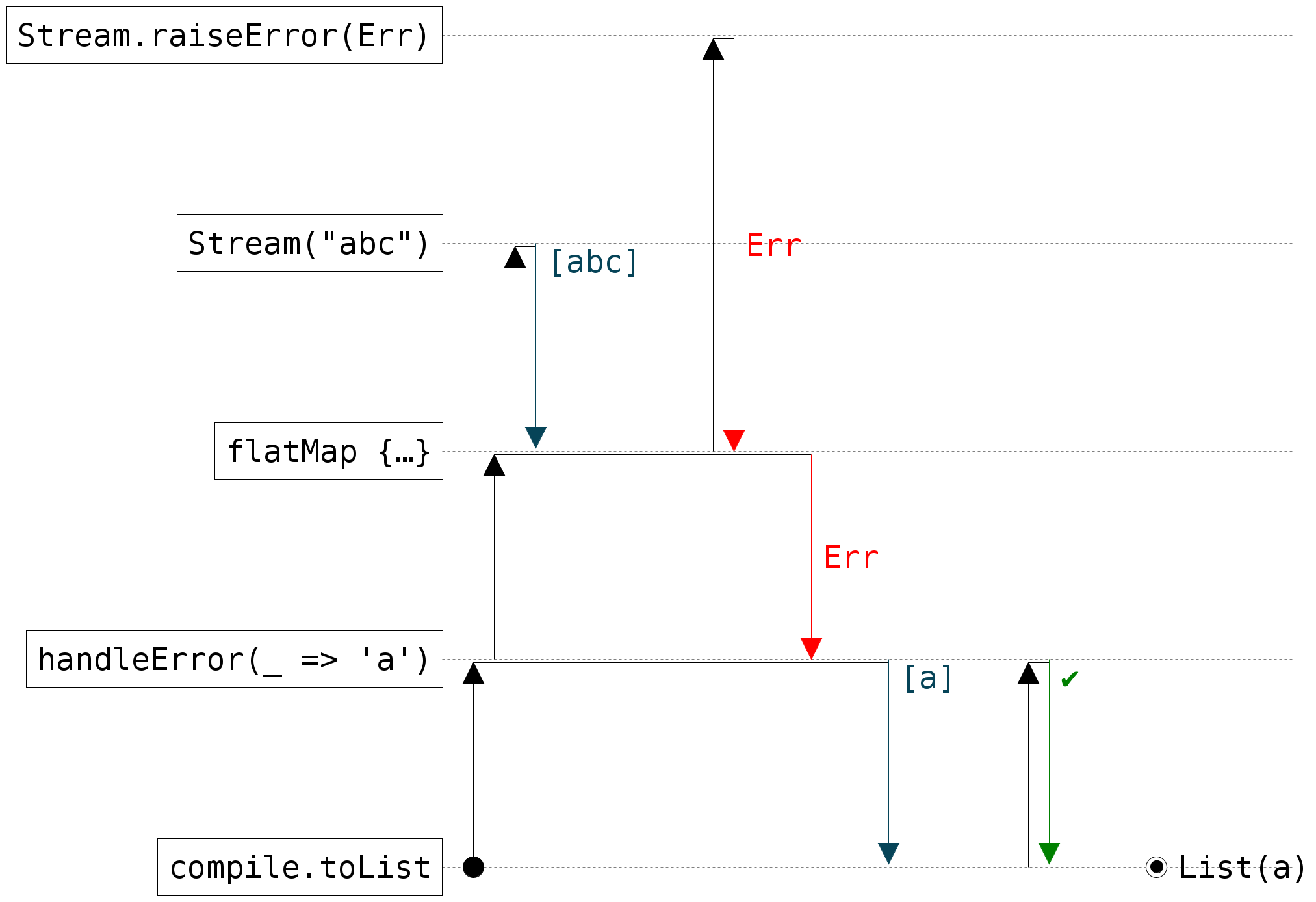
bracket
Stream('a', 'b', 'c')
.flatMap { x =>
Stream.bracket(IO(s"<$x"))(_ => IO(s"$x>").void)
}
.compile
.toList

bracket (with chunks)
Stream('a', 'b', 'c')
.flatMap { x =>
Stream.bracket(IO(s"<$x"))(_ => IO(s"$x>").void)
}
.compile
.toList
